Let's be honest... Sometimes as developers we need to change that one line of code. But we are not sure if it will break part of our site.
We have two options. We can work on our development environment, or we can make our changes to the production site.
Development vs. Production
There are many valid reasons why you might edit your website in a production environment. One that I run into is there is a slight difference between production and development. That difference is the source of a problem. But, there are other reasons also.
The site may be too small to have a regular development environment. Creating a copy of the site locally or on another server takes time.
We can rationalize why it might make sense edit your live site.
However, if you are going to, there are some rules you should follow and some tips to help you do it right.
Basic Rules
Yes.. I edit code on a production environment sometimes.
But when I do, I follow a few basic rules!
1. Backup! Backup! Backup!
The first rule of editing your production site is to be cool and back it up first! I will say it again back up your website!
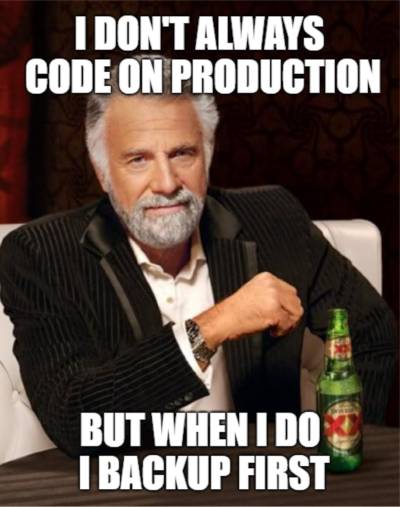
I personally recommend using Akeeba Backup. I use it on almost all of my Joomla websites.
2. Don't break what you can't fix.
There are some things in Joomla that are harder to fix than others. If you don't know what DROP TABLE
does, don't change it. It deletes a table from your database. Even if you undo your edit, the table is still gone.
Joomla doesn't work so well with a corrupted database. It doesn't work much better with parts deleted.
You need to know what is affected by what you are changing. Which takes us to our next rule.
3. Know what is downstream.
If you change a variable or a foreach loop or anything, it can change more than you are trying to effect.
You need to know the full extent of what you are changing. And to be able to know that you must know what other parts of the code depend on what you are modifying.
4. Don't show everyone your code.
While you are making changes or troubleshooting, visitors should not see errors, broken parts of your site, or any evidence of the changes you are making.
There are a few ways to do this.
You can restrict your code to only run for your IP address. I like this method better than restricting it to admin users.
Helpful Code Snippets
In light of rule number four, I have three code snippets you may find helpful.
Restrict your code to admins.
The following snippet will only execute your edit if the user is logged in with a viewing access level of Special or greater.
$user = JFactory::getUser();
$is_admin = $user->authorise('core.admin');
if ($is_admin) {
error_reporting(-1);
// Code that only admins can see.
}
On production you should have the error reporting in Joomla set to none. However, seeing PHP errors is still important as a developer. To get around this I include error_reporting(-1)
in the if statement. This lets me see errors without showing them to everyone.
Restrict your code to your IP.
Sometimes you have code you need to be executed when you are not logged in. In that case, you can use this snippet.
$app = JFactory::getApplication();
$ip = $app->input->server->get('REMOTE_ADDR', '');
$is_dan = false;
if ($ip === 'xxx.xxx.xxx.xxx') {
$is_dan = true;
}
if ($is_dan) {
error_reporting(-1);
// Code that only you can see.
}
I generally prefer this over the admin method.
You will need to replace xxx.xxx.xxx.xxx
with your IP address.
Also don't be lazy! Rename the variable $is_dan
. Unless your name is also Dan, then you can use it. However, the rest of you can go make your own variable. $is_dan
is mine!
Restrict a directory to your IP.
Sometimes you need to restrict access to a directory or the entire site for a moment. You can do this and still have access yourself.
This snippet redirects all traffic except your IP to a page called update.html
. This can be a static page in your site's root directory.
RewriteEngine On
RewriteBase /
<If "!-R 'xxx.xxx.xxx.xxx'">
RewriteCond %{REQUEST_URI} !^/update\.html
RewriteCond %{REQUEST_URI} ^(.*)$
RewriteRule ^(.*)$ https://www.example.com/update.html?path=%1 [R=302,L]
</If>
Make sure to replace xxx.xxx.xxx.xxx
with your IP. Also replace https://www.example.com
with your scheme and domain.
If you chose to change the name of update.html
, be sure to change it both times it occurs.
Did you notice that I append the user's relative path as a query string at the end of the redirect URL?
I do that for a reason. On the update.html
page use JavaScript to pull value from the query string with the path
key. I then append the path to the root domain on a button to return to the page they were viewing.
The JavaScript looks like this.
window.onload = function() {
var urlParams = new URLSearchParams(window.location.search);
path = urlParams.get('path');
if (typeof path !== 'undefined' || path !== null) {
document.getElementById("back-to-page-btn").href = "https://www.example.com"+path+"";
}
}
I simply throw this in a pair <script>
tags in the document head.
Later on the page, I create a link to go back to the page user was on.
<a id="back-to-page-btn" href="https://www.example.com">Try going back to page</a>
The JavaScript appends the relative path to the end of the URL and the overwrites the value of the href
attribute.
It is pretty simple, but it makes the user experience much better.
My Final Thoughts
When it comes to making changes to the code of your Joomla website, I understand the pressure to just try and change something without moving to a development environment. I have been in your shoes.
That is why I wrote and shared these rules and snippets of code. I have used every one of them myself.
They are meant to help make your job easier and keep your website looking professional even if you are making changes under the hood.